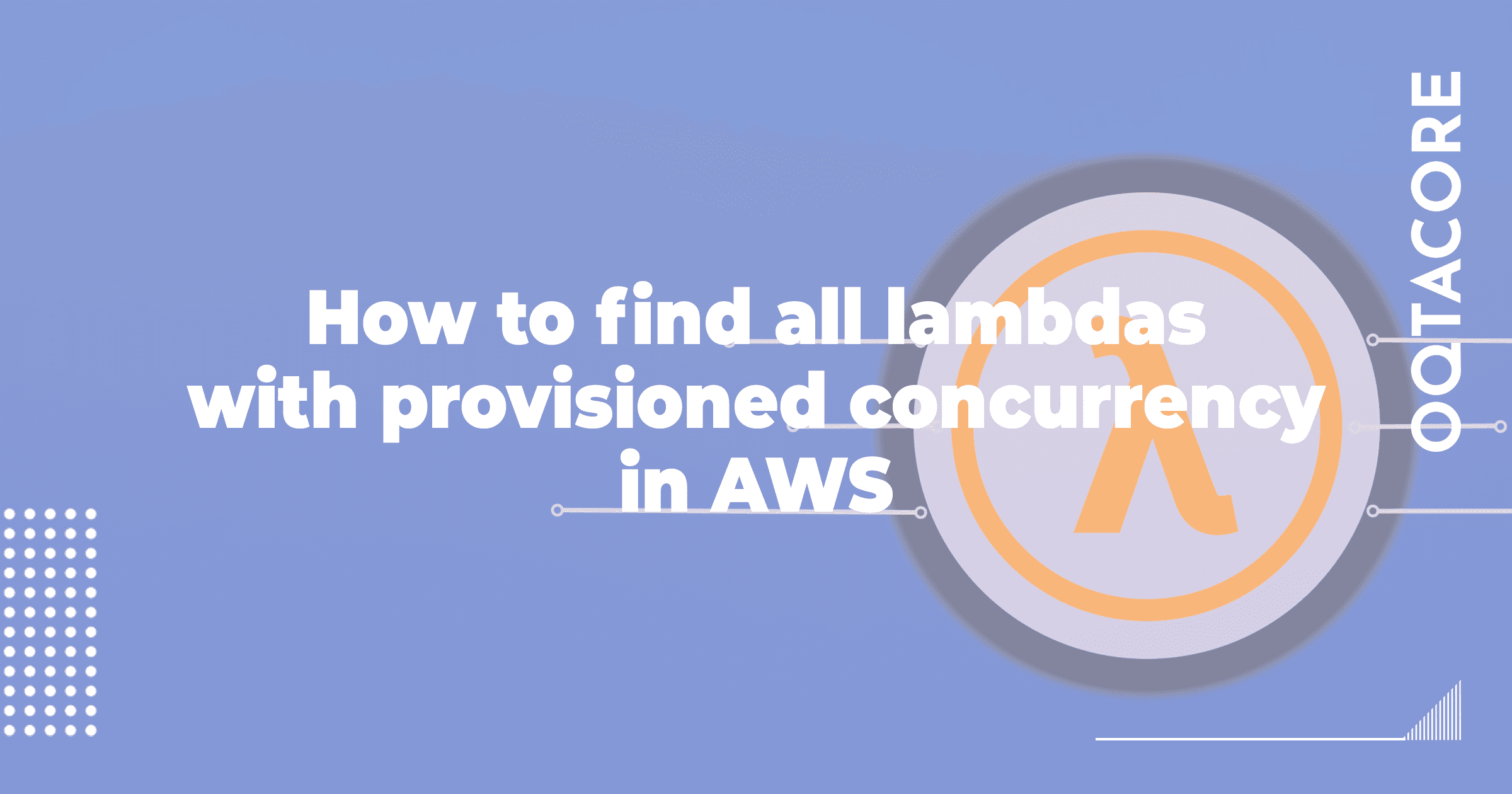
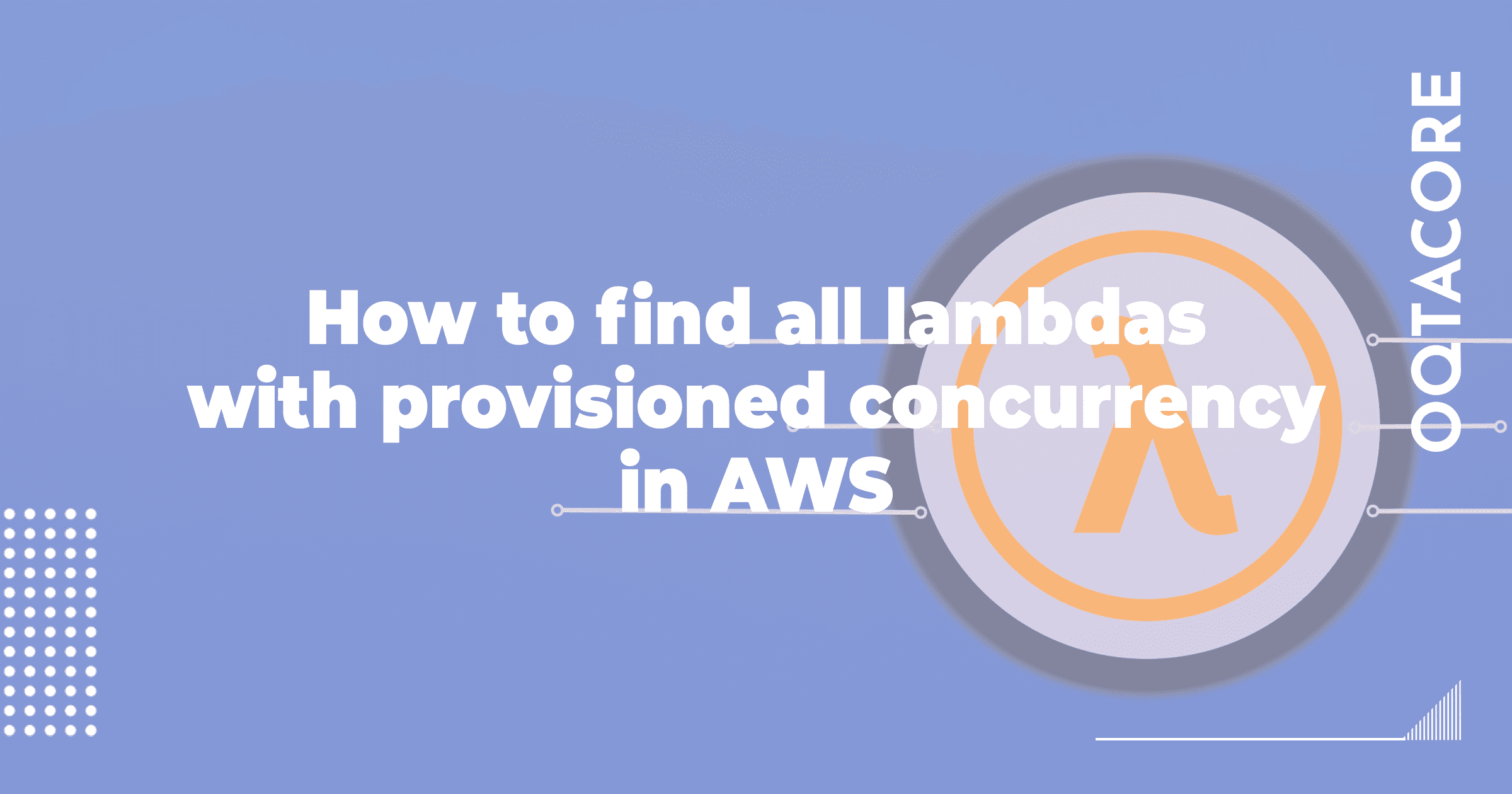
AWS lambdas are great for many various use cases. They are cheap (if used correctly), fast and easy to maintain. A lambda once written can easily be launched again, unlike most other code that you have on your local disk.
But there are some downfalls in lambdas – one is that they need some warm-up time if they are not used often. By default, your lambda code is not loaded into the memory of the worker that runs this lambda. And when an unused lambda is invoked, it first needs to be loaded into a container and started. Such a situation is called a cold start, and a cold start takes 700ms to 3 seconds from our experience.
After a cold start, a container stays warm for about 30-40 minutes, and then a cold start will happen again.
Source: AWS
But what if you need your lambda to be used rarely, but each response should be very quick? For example, it might be your newly developed startup project with a marketplace page for which some lambda processes user requests. Until you get enough users to keep your lambdas always warm, you might need to keep them warm yourself so that users do not experience multi-second load times while accessing the marketplace. Otherwise, your conversion rates will be a disaster.
Source: Cloudflare
And AWS thought of that – they allow you to keep your lambdas warm. It is called provisioned concurrency (do not mistake it with reserved concurrency which is absolutely different).
Provisioned concurrency keeps containers with your lambda warm, and it will take just a few tens of milliseconds to start the lambda.
However, take note: the provisioned concurrency is not free. And oftentimes you just forget which lambdas have it enabled, and checking each lambda can take 30 seconds even if you know where to check – that’s because provisioned concurrency settings are just buried too deep in the lambda settings and also require checking each version of lambda separately… Extremely bad UX.
So when we at OQTACORE faced an issue of needing to check where we have provisioned concurrency enabled, there was no better way than to write a simple lambda to fight provisioned concurrency of other lambdas 🙂
Here is the code:
const AWS = require(‘aws-sdk’);
const lambda = new AWS.Lambda(); exports.handler = async (event) => { let promises = []; const allLambdas = await getAllLambdas(); const resultArr = []; for (let i = 0; i < allLambdas.length; i++) { promises.push(checkConfig(allLambdas[i].FunctionName, resultArr)); } await Promise.all(promises); return resultArr; } async function getAllLambdas() { let nextMarker; let allLambdas = []; do { var params = { Marker: nextMarker, MaxItems: 10 }; let result = await lambda.listFunctions(params).promise(); allLambdas = […allLambdas, …result.Functions]; nextMarker = result.NextMarker; } while (nextMarker != null); return allLambdas; } async function checkConfig(funcName, resultArr) { let retries = 5; //sometimes listProvisionedConcurrencyConfigs() crashes while (retries– > 0) { try { var params = { FunctionName: funcName }; const conf = await lambda.listProvisionedConcurrencyConfigs(params).promise(); if (conf.ProvisionedConcurrencyConfigs.length > 0) { resultArr.push(conf); } break; } catch (ex) { console.log(ex) } } } |
This code will return you an array of lambdas that have provisioned concurrency enabled. Please note that this works only in the same region of the lambda! You can modify the code yourself to process all regions that you need, or just deploy this code in every region where you have lambdas.
If you are looking for software developers who know cloud and blockchain, make sure to check OQTACORE.