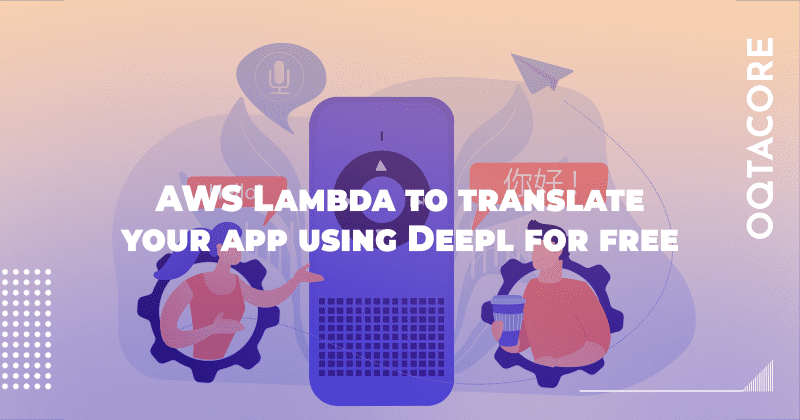
Imagine you have a modern app that you would want to translate to other languages quickly using machine translation. Using this code, you can translate your app to all languages in minutes. For free.
Chances are, you do not want to spend $200 per language. You just want to quickly rollout your app to all possible locales, see the engagement and metrics, and then decide if you want to move forward.
Here is AWS Lambda code that takes a file named “app_main.en.json” from S3 and creates translated copies of it named “app_main.fr.json”, “app_main.de.json”, etc.
You will need to create a free account at Deepl to get an API authentication key.
const AWS = require('aws-sdk'); const S3 = new AWS.S3(); const https = require('https'); const SOURCE_LOCALE = 'en'; // Replace with the source locale of your i18next JSON file const TARGET_LOCALES = ['de', 'fr', 'es']; // add or remove languages as needed const FILE_NAME = 'app_main'; const BUCKET = 'your bucket'; const DEEPL_API_KEY = 'Register at Deepl to get your API authentication key'; exports.handler = async (event) => { // Retrieve the i18next JSON file from S3 const params = { Bucket: BUCKET, Key: `${FILE_NAME}.${SOURCE_LOCALE}.json` }; const data = await S3.getObject(params).promise(); const i18nData = JSON.parse(data.Body.toString('utf-8')); // Loop through each target language and translate the i18n data using DeepL API const translations = {}; const savePromises = []; for (const language of TARGET_LOCALES) { const translatedJson = {}; const promises = Object.entries(i18nData).map(async ([key, value]) => { translatedJson[key] = await translate(value, language); }); await Promise.all(promises); const s3Params = { Bucket: BUCKET, Key: `${FILE_NAME}.${language}.json`, Body: JSON.stringify(translatedJson), ContentType: 'application/json' }; const savePromise = S3.putObject(s3Params).promise(); savePromises.push(savePromise); } await Promise.all(savePromises); return { statusCode: 200, body: JSON.stringify(translations) }; }; // Helper function to translate the i18n data using DeepL API function translate(text, targetLanguage) { return new Promise((resolve, reject) => { const apiKey = DEEPL_API_KEY; const options = { hostname: 'api-free.deepl.com', path: '/v2/translate', method: 'POST', headers: { 'Content-Type': 'application/x-www-form-urlencoded' } }; const request = https.request(options, (response) => { let chunks = []; response.on('data', (chunk) => { chunks.push(chunk); }); response.on('end', () => { const translation = JSON.parse(Buffer.concat(chunks).toString()); console.log(JSON.stringify(translation)); resolve(translation.translations[0].text); }); }); request.on('error', (error) => { reject(error); }); const data = new URLSearchParams(); data.append('text', text); data.append('auth_key', apiKey); data.append('source_lang', SOURCE_LOCALE); data.append('target_lang', targetLanguage); request.write(data.toString()); request.end(); }); }